DevOps E4DRR- Code patterns, project management, infrastructure, workflow
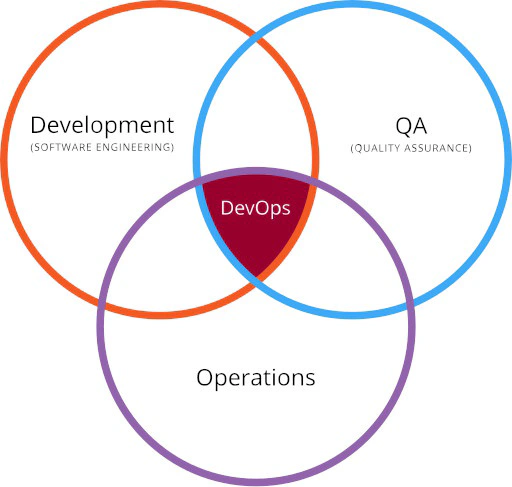
Introduction
This document outlines patterns and practices for development related to E4DRR project, covering code patterns, project management, infrastructure, and workflow management.
1. Code Patterns
In this section, we discuss code patterns that ensure robust, maintainable, and scalable codebases. These patterns include best practices for exception handling, code structure, logging, and testing using Python.
1.1 Exception Handling and Logging
Effective exception handling and logging ensure that errors are captured, diagnosed, and resolved efficiently. Below is a Python example implementing a function with proper try-except blocks and logging enabled.
import logging
# Configure logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def divide_numbers(a, b):
try:
result = a / b
logging.info(f"Division successful: {a} / {b} = {result}")
return result
except ZeroDivisionError as e:
logging.error(f"Error: Division by zero - {e}")
except Exception as e:
logging.error(f"An unexpected error occurred: {e}")
# Test the function
divide_numbers(10, 2)
divide_numbers(10, 0)
1.2 Unit Testing with Pytest
Testing is an essential part of any scalable system. Here’s an example of a unit test using pytest for the divide_numbers
function, with code coverage to ensure all branches are tested.
import pytest
from my_module import divide_numbers # Assuming your function is stored in a module
def test_divide_numbers_success():
assert divide_numbers(10, 2) == 5
def test_divide_by_zero():
assert divide_numbers(10, 0) is None
# Running tests
if __name__ == "__main__":
pytest.main()
1.3 Code Coverage
Ensure that the code coverage tool is set up in your CI/CD pipeline to guarantee that each function is properly tested. You can achieve this with pytest-cov:
pytest --cov=my_module tests/
2. Project Management
Project management is based on Agile methodologies. The key to maintaining agility is in frequent iterations, collaboration, and timely adjustments based on feedback.
2.1 Agile Project Management with Asana
In this project, we adopt Agile principles, specifically a bi-weekly sprint framework using Asana. This allows us to create clear sprint goals, track tasks, and adapt to any emerging requirements.
Sprint Planning
- Backlog: We maintain a backlog of tasks/features to work on, prioritized based on business needs and technical feasibility.
- Sprint Planning: Every two weeks, we select tasks from the backlog to be completed during the sprint.
- Daily Standups: Team members provide updates and report any blockers.
- Sprint Review: At the end of the sprint, we review completed tasks and gather feedback from stakeholders.
- Retrospective: Reflection on what worked and what didn’t to improve future sprints.
With Asana, we create tasks and subtasks under each sprint, set deadlines, assign team members, and track progress in real time.
3. Infrastructure
Building scalable infrastructure is essential for handling large-scale data and tasks. Below, we describe the tools used for distributed computing, infrastructure as code, and web processing services.
3.1 Distributed Computing with Coiled and Dask
We use Coiled to manage Dask clusters in Python, which enables efficient parallel computing. Coiled provides a managed environment for creating and running Dask clusters on the cloud, handling the complexity of scaling computations.
Example configuration for setting up a Dask cluster with Coiled:
import coiled
from dask.distributed import Client
# Create a Coiled cluster
cluster = coiled.Cluster(
software="my-environment",
n_workers=10,
worker_memory="4GiB",
)
client = Client(cluster)
This setup allows for distributed computation across multiple workers, making it ideal for data-intensive tasks.
3.2 Infrastructure as Code with Terraform
To provision and manage cloud infrastructure, we use Terraform. It allows us to define infrastructure as code, which makes deployment repeatable and version-controlled. Here’s an example of a Terraform configuration to provision resources on Google Cloud Platform (GCP):
provider "google" {
project = "my-gcp-project"
region = "us-central1"
}
resource "google_compute_instance" "default" {
name = "my-instance"
machine_type = "e2-medium"
zone = "us-central1-a"
boot_disk {
initialize_params {
image = "debian-cloud/debian-10"
}
}
network_interface {
network = "default"
}
}
3.3 Web Processing with GCP Cloud Run
For deploying web-based processing services, we leverage GCP Cloud Run, a fully managed platform that scales containerized applications automatically. This allows us to deploy our services in a serverless environment, reducing operational complexity and costs.
4. Workflow Management
Managing complex workflows in large systems requires specialized tools, especially when dealing with tasks like impact-based forecasting, where various datasets and models must be integrated.
4.1 ECMWF Workflow System
The European Centre for Medium-Range Weather Forecasts (ECMWF) uses an advanced workflow management system (ecFlow/pyflow)[1] to coordinate complex meteorological and environmental forecasting tasks. This system handles diverse data inputs and manages the dependencies between different forecasting models and datasets.
4.2 Impact-Based Forecasting with Prefect
For Impact-Based Forecasting (IBF), which focuses on predicting the potential impacts of weather events such as flooding and extreme temperatures, it is critical to use a system capable of managing multiple workflows and datasets simultaneously. We utilize Prefect, an open-source, managed service that orchestrates all the necessary components for IBF. The development of Prefect, originally designed for financial risk management [2], provides significant motivation for its use in routine impact-based forecasting and positions it as a valuable tool from a risk assessment perspective. This approach aligns well with prevailing directives on weather and climate risk assessment, which advocate for conducting risk assessments whenever possible with whatever data is available.
Key Features of Prefect:
- Data Ingestion: Automatically ingests multiple data products needed for risk assessment, including meteorological data, GIS data, and historical impact data.
- Task Orchestration: Manages the sequence of tasks, ensuring data dependencies are resolved before running the next step.
- Scalability: Can handle large-scale data processing required for national or regional-level impact forecasting.
Prefect’s open-source nature also allows for custom integrations and the ability to run it on both local infrastructure and cloud environments.
By integrating these best practices and technologies, we ensure that our development process is both scalable and robust, capable of handling the growing complexity of modern applications.
Refrences